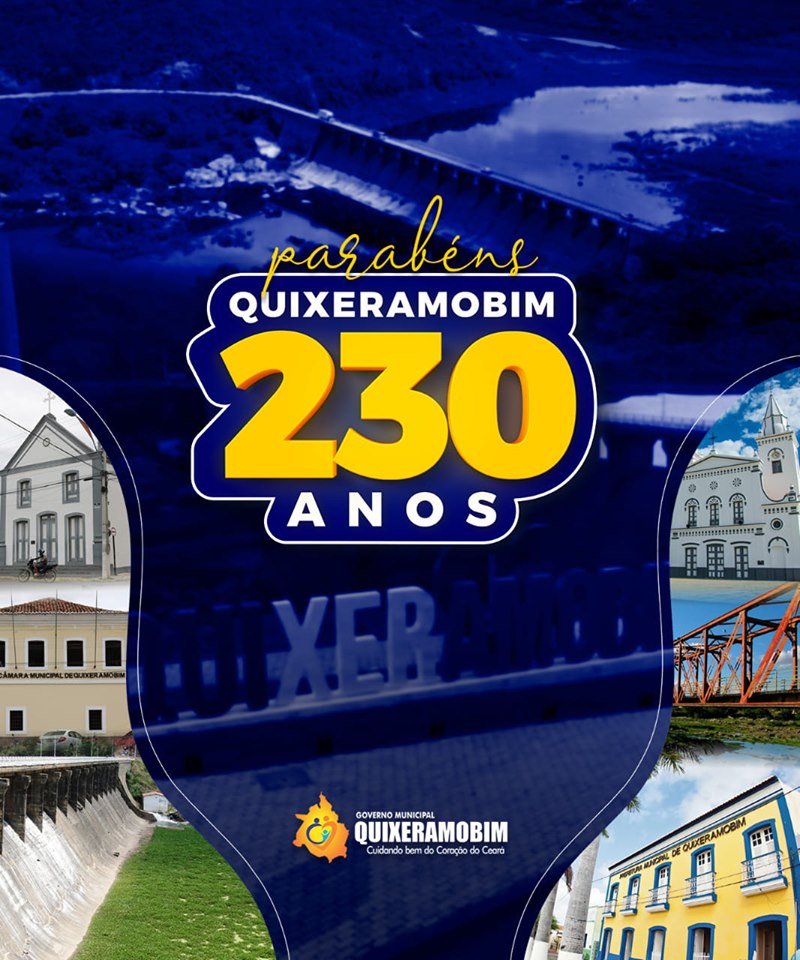
Região Central: O município celebra hoje, 14 de agosto, 230 anos de emancipação política.
Quixeramobim é um município do estado do Ceará, a 203 km de Fortaleza. É a segunda maior cidade do sertão central, com uma população de 75.565 habitantes. O volume 16º da Enciclopédia dos Municípios Brasileiros conta que, segundo a tradição, os primitivos habitantes da região eram os índios quixarás. Os primeiros civilizados que penetraram aquelas terras vieram do Jaguaribe, seguindo o Rio Banabuiú. Eram membros das famílias Correia Vieira e Rodrigues Machado, que ali se estabeleceram com fazendas de criar. A povoação parece ter nascido precisamente dessas fazendas.
Quixeramobim é um município de uma grande riqueza cultural, terra de filhos ilustres, como Antônio Conselheiro (tendo nascido em Quixeramobim em 13 de Março de 1830) líder da guerra de Canudos e Fausto Nilo, arquiteto e grande compositor da música popular brasileira; terra onde se deu início a Confederação do Equador e muitas páginas tem escrito na história do Brasil, como a primeira comunidade a ter sua energia gerada por Biodielsel, a Serrinha de Santa Maria. Encravado no Vale Monumental do Ceará, onde a paisagem de monólitos e caatinga atrai os visitantes, o Município Coração do Ceará se destaca pela existência dos sítios arqueológicos e por seus atributos históricos e culturais.
Pontos Turísticos e de visitação
Barragem de Quixeramobim
Casa da Câmara e Cadeia
Casa de Antônio Conselheiro
Centro geodésico do Ceará
Memorial Antônio Conselheiro
Pedra do Letreiro (sítio arqueológico)
Igreja de Nosso Senhor do Bonfim
Igreja Matriz de Santo Antônio
Paço municipal
Ponte metálica